Python Win32serviceutil Install Service
News about the dynamic, interpreted, interactive, object-oriented, extensible programming language PythonIf you are about to ask a 'how do I do this in python' question, please try, or the #python IRC channel on FreeNode.Please don't use URL shorteners. Hi, I'm trying to run a dummy Python script as a service in Windows 7.
It just writes the current time to a file in disk. I've debugged and solved a similar problem.The cause was that the service runs as a different user (the SYSTEM or SERVICE user, IIRC) when it is started normally, and that user also has to have the environment set up properly for python to work. In particular, it has to have the PATH environment variable (and possibly also PYTHONPATH and/or PYTHONHOME) set up so that the python executable is found and executes properly. EDIT: This probably means copying settings from user environment variables to system environment variables under System Properties = Advanced = Environment Variables.If this fails to fix the problem, see for how to debug a service, this goes for python services as well. Windows services are worse than hell.
Every single part of the puzzle is absolutely vital, or something won't work right. In your case, I think your issues is your SvcDoRun method.

Look at mine which does work (last i checked): def SvcDoRun(self):' Run the service, starting our CommServer which handles all other necessary modules.' 'self.server = CommServerself.server.run(daemon=True)self.timeout = FREQUENCY.
1000 # frequency seconds (timeout is in milliseconds)rc = Nonewhile rc!= win32event.WAITOBJECT0:# Wait for stop signal, loop on timeoutrc = win32event.WaitForSingleObject(self.hWaitStop, self.timeout)The crucial part you're missing is the while loop checking rc = win32event.WAITOBJECT0. This lets the service handle stopping your script if necessary and keeps it from locking up, and i think that's your issue. It times out because you never give the service control to tell it that it's started up. In my case, my server.run function runs a tcp server in the background, on another thread, and this is key. Any of your application logic either needs to include sleeps and this win32event loop, or run in it's own thread that can handle being killed (the latter is the more stable option from my findings).Also on another note, in the SvcStop method, i've found that it's useful to add a sys.exit(0) call as the last line of the function, as without this i seemed to get a lot of false errors when stopping the service.
Now any like me has a need to schedule their things (in production) every once in awhile. Usually this is not a problem - Unix / Linux cron jobs handle this nicely - but for a client or job that runs on a Windows server - the built-in 'Scheduled Tasks' just never really cut it for me - in fact, I've always though that it was pretty Mickey-Mouse and not super reliable (just my opinion - don't flame me, you wily Windows Dudes - I know that there are 1,000 ways to skin a cat, here's mine!).It honestly gave me the creeps to put production stuff on there that NEEDED to be run every X minutes, hours, etc.
Besides, making a batch file to fire off a python scripts feels so. Well, unpolished - and pretty much of a lazy hack that made it into prod.Disclaimer: Hey, I love hacks - they make the IT world go around, but I don't like delivering shit to clients that have loose feeling triggers like that. Especially, if some smart ass IT dickface (who COULDN'T pull off what I did, or else I wouldn't have been paid to do it) is going to dig into them one day and bad mouth me about it (rightfully so).Besides, I'll find you!:)Enough blather - here is the whole script. Modify and distribute where you see fit - parts of it were written by others that I can't recall (so if its you - please accept my apology and an expired gift card). ### Run Python scripts as a service example (ryrobes.com)### Usage: python aservice.py install (or / then start, stop, remove)import win32serviceimport win32serviceutilimport win32apiimport win32conimport win32eventimport win32evtlogutilimport os, sys, string, timeclass aservice (win32serviceutil.
Python Windows Service Control
ServiceFramework ):svcname = 'MyServiceShortName'svcdisplayname = 'My Serivce Long Fancy Name!' svcdescription = 'THis is what my crazy little service does - aka a DESCRIPTION!
Def init ( self, args ):win32serviceutil. init ( self, args )self. HWaitStop = win32event.
CreateEvent ( None, 0, 0, None )def SvcStop ( self ):self. ReportServiceStatus (win32service. SERVICESTOPPENDING )win32event. SetEvent ( self. HWaitStop )def SvcDoRun ( self ):import servicemanagerservicemanager. LogMsg (servicemanager. PYSSERVICESTARTED, ( self.svcname, ' ) )#self.timeout = 640000 #640 seconds / 10 minutes (value is in milliseconds)self.

Timeout = 120000 #120 seconds / 2 minutes# This is how long the service will wait to run / refresh itself (see script below)while 1:# Wait for service stop signal, if I timeout, loop againrc = win32event. WaitForSingleObject ( self. HWaitStop, self. Timeout )# Check to see if self.hWaitStop happenedif rc win32event.
WAITOBJECT0:# Stop signal encounteredservicemanager. LogInfoMsg ( 'SomeShortNameVersion - STOPPED!' ) #For Event Logbreakelse:#Ok, here's the real money shot right here.#actual service code between reststry:filepath = 'C: whereever myREALpyworktobedone.py'execfile (filepath ) #Execute the scriptincfilepath2 = 'C: whereever MOREREALpyworktobedone.py'execfile (incfilepath2 ) #Execute the scriptexcept:pass#actual service code between restsdef ctrlHandler (ctrlType ):return Trueif name 'main':win32api. SetConsoleCtrlHandler (ctrlHandler, True )win32serviceutil. HandleCommandLine (aservice )# Done!
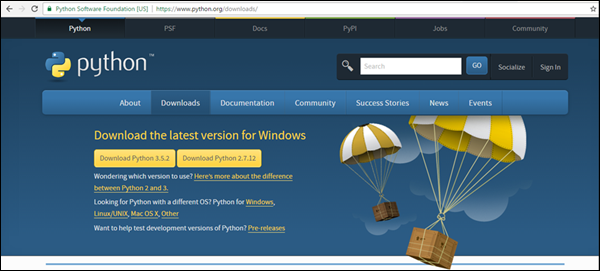
Lets go out and get some dinner, bitches!As you can see above between the actual service code between rests text - THAT'S the real meat of this script - since that is the ACTION that will be executed within the service with each iteration (regardless of the timing).So it doesn't matter if you're:. Running some Python ETL processes. Scraping some data off the web. Sending emails and reading IMAP accounts. Updating a Data warehouse. EMail your Grandmother the latest news from Blabbermouth.netHell, it can be and do virtually anything!
Hey, its YOUR service after all.Don't want to Cut-N-Paste? I don't blame you - download. Questions, Comments, Corrections?
Comment me below!(especially corrections - sometimes things get mangled in cut-n-paste). The script above is fine- it’s the windows python bits that need help. It took two fixes for me to get this working1/ make sure your python has got an an entry in the registry. This is vital for step 2/ — See handy script here2 / install the windows compiled versions of pypi win libray – search for the right build for your version and bit size (32 or 64). If you don’t do the first step, the installer will throw up, saying your python isn’t in the registryOnce those two steps were done, the service ran happily.